Optimizing Word Hunt with a Radix-Tree Based Pathfinding Algorithm
Word Hunt, a popular game in iMessage, involves a grid of letters where players swipe through to create words and score points. The longer the word, the more points one can score. However, this seemingly simple game can become highly competitive, as players compete to find longer and more complex words. To level the playing field, I've developed a bot that uses a radix-tree based pathfinding algorithm to find the optimal words in the grid. In this article, I will explore the algorithm and its implementation in depth.
Understanding Tries and Radix Trees
Before diving into the algorithm, it is essential to understand what tries and radix trees are. A trie, also known as a prefix tree, is a tree-like data structure that stores a dynamic set or associative array where the keys are usually strings. Each node in the trie represents a character in a string, and nodes are connected based on the order of the characters in the strings. Tries are efficient for solving problems that involve searching through large dictionaries, as they reduce the complexity of lookups.
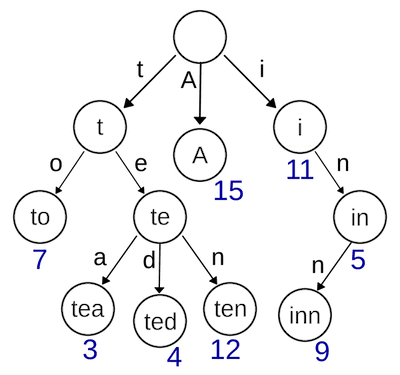
A radix tree, or a compressed trie, is an optimized version of a trie that eliminates redundancies in the structure by combining nodes with single children. This compression reduces the memory usage and speeds up lookups.
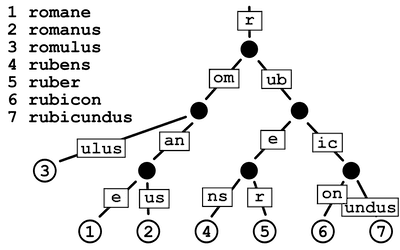
The Algorithm:
The radix-tree based pathfinding algorithm designed for the Word Hunt game works as follows:
- Begin at the first letter of a word in the grid.
- Use a radix tree containing a dictionary of valid words to identify possible next letters based on the grid's layout.
- Traverse the grid using a depth-first search (DFS) approach while considering the constraints of the game, such as not using the same letter twice.
- Continue the traversal until a valid word is formed or the path is exhausted.
- Repeat steps 1-4 for all possible starting points in the grid.
- Store and display the highest-scoring words found.
Benefits of the Algorithm:
By utilizing a radix tree, the algorithm offers several benefits, including:
Efficient memory usage: A radix tree compresses the data structure, reducing memory overhead when compared to a standard trie. Faster lookups: The compressed structure enables faster lookups by minimizing the number of nodes traversed during searches. Scalability: The algorithm can handle larger dictionaries and grids, enabling it to find complex words with high point values.
Conclusion:
The radix-tree based pathfinding algorithm provides an efficient and effective solution for solving the Word Hunt game. By leveraging the benefits of a radix tree, the algorithm optimizes memory usage and lookups while maintaining scalability. With the help of this bot, players can now compete against more skilled opponents and enjoy a new level of challenge in the Word Hunt game. In other words, I can now beat my girlfriend at Word Hunt.
To look into the source code, please visit github.com.